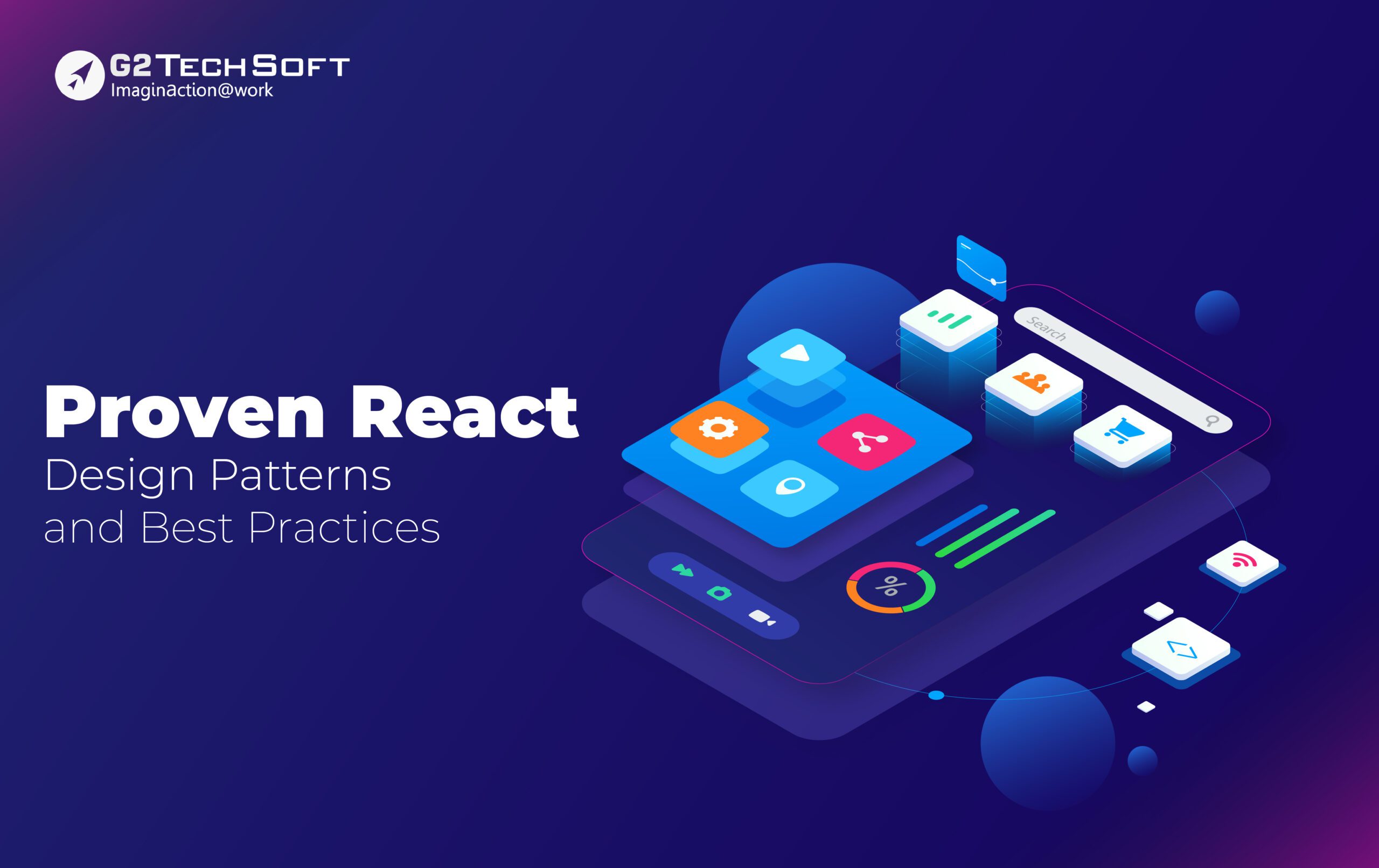
Proven React Design Patterns And Best Practices
Traditional web development used to be complex, but the introduction of React has greatly simplified the process. The reusable components and extensive ecosystem of React make it efficient and user-friendly.
The React ecosystem offers various tools, some designed to meet various development needs and others to solve specific issues. React Design Patterns provide quick and reliable solutions to common development challenges.
In ReactJS, development companies employ numerous design patterns, each serving a distinct purpose in a project. This article discusses some essential design patterns that React developers should be familiar with.
Understanding React.js Design Patterns:
ReactJS design patterns provide effective solutions to common challenges that developers encounter during the software development process. These patterns are reusable, which helps streamline the codebase and reduce the overall size of the application. By using React design patterns, developers can avoid code duplication when sharing component logic.
Complications are inevitable in any software development project. However, design patterns offer reliable solutions that can simplify these complexities, making the development process more efficient. Additionally, they promote writing clean, easy-to-read code, enhancing both maintainability and readability.
Advantages Of Using Design Patterns In React Development:
These advantages significantly enhance the effectiveness of React development and will make you familiar with the React Design Patterns.
Reusability:
React Design Patterns offer reusable templates that permit you to build components efficiently. This approach saves time and effort in application development, as you don’t have to start from scratch with each new project.
Collaborative Development:
React facilitates collaborative software development by enabling multiple developers to work on the same project. Design patterns provide a structured framework that helps manage these collaborative efforts effectively, preventing potential issues and improving project coordination.
Scalability:
Design patterns permit organized and modular code, making it effortless to maintain and scale even large applications. Each component operates independently, so changes to one do not affect others, simplifying the scaling process.
Maintainability:
Design patterns offer systematic solutions to common development challenges, making coding simpler and more maintainable. This is particularly beneficial for large React projects, as modularity ensures that changes to one part of the code do not impact the entire application, promoting easier maintenance.
Efficiency:
React’s component-based design, coupled with the Virtual DOM, ensures faster loading times and quick updates, enhancing overall application efficiency and user experience. Design patterns like memoization further improve performance by caching expensive rendering results and limiting unnecessary re-renders.
Flexibility:
React’s component-based architecture allows for easy modifications and experimentation with different component combinations to create unique solutions. This flexibility is crucial for developing user interfaces that stand out in the marketplace. React’s lack of strict guidelines enables developers to mix and match various approaches, fostering creativity and innovation.
Consistency:
Following React design patterns creates a dependable and transparent application. Uniformity enhances the user experience and simplifies navigation, which can increase user engagement and boost revenues.
In a nutshell, understanding and utilizing React Design Patterns can significantly enhance the development process, making your applications more reusable, collaborative, scalable, maintainable, efficient, flexible, and consistent. These benefits position your business for success in the competitive digital marketplace.
Pivotal React Design Patterns With Pros And Cons Each Developer Must Know
Higher Order Component (HOC) Pattern:
A HOC is an advanced technique in React for reusing component logic. A HOC is a function that takes a component as an argument and returns a new component with added functionality. This pattern allows you to encapsulate behavior that can be shared across multiple components without repeating code.
Pros:
- Reusability: HOCs permit you to reuse component logic across distinct components without duplicating code.
- Separation of Concerns: By abstracting common logic into HOCs, you can keep your components focused on their primary purpose.
- Enhanced Functionality: HOCs can add new behavior or data to components, enhancing their functionality without modifying the components themselves.
Cons:
- Prop Conflicts: When multiple HOCs are used, there can be conflicts with prop names, making debugging more difficult.
- Complex Debugging: HOCs can make the component hierarchy more complex, complicating the debugging process.
- Performance Overhead: Each HOC adds a layer of abstraction, which can impact performance, especially if multiple HOCs are nested.
Provider Pattern:
The Provider pattern is a design approach in React that uses the Context API or Redux to manage state and make data accessible to multiple components without prop drilling. A Provider component is placed at the top of the component tree and passes down data to its children via context, avoiding the need to pass props through many layers.
Pros:
- Centralized State Management: It provides a central place to manage the state, improving the organization and maintainability of your application.
- Avoids Prop Drilling: By eliminating the need to pass props through multiple levels, it simplifies component structures and makes data access easier.
- Global Access: Components can access shared data from anywhere in the component tree, promoting consistency and reducing duplication.
Cons:
- Performance Issues: Overuse of the Provider pattern, particularly with frequently changing data, can lead to performance problems.
- Complexity: Setting up and managing the Provider pattern adds complexity to the codebase, especially for developers unfamiliar with React Context or Redux.
- Overhead for Simple Apps: For small applications, the complexity of implementing a Provider pattern might be unnecessary, adding more overhead than benefit.
Container/Presentational Pattern:
This pattern separates the logic (container components) from the view (presentational components) in React applications. Container components manage state and business logic, while presentational components focus on rendering data and user interfaces.
Pros:
- Clear Separation of Concerns: This pattern divides the application into distinct layers, making it easier to manage and understand.
- Reusability: Presentational components shall be reused in distinct parts of the application since they are not related to particular business logic.
- Testability: Presentational components are easier to test because they are primarily concerned with rendering and not with managing states or logic.
Cons:
- Boilerplate Code: Implementing this pattern can introduce additional boilerplate, as you need to create both container and presentational components.
- Complexity: For small applications, this separation might add unnecessary complexity without significant benefits.
- Transition Issues: Stateless functional components may need to be rewritten as class components or vice versa to fit into this pattern, which can be cumbersome.
Compound Pattern:
This pattern permits distinct interdependent components to share logic and state, working together to finish a task. This pattern enables a parent component to communicate with and control its children in a coordinated manner, providing a flexible API for managing component interactions.
Pros:
- State Management: Compound components maintain an internal state that is shared among child components, simplifying state management.
- Flexibility: This pattern provides a flexible API for child components to interact with the parent component and with each other.
- Encapsulation: Complex interactions are encapsulated within a single component, making the logic easier to manage and understand.
Cons:
- Limited Component Stacking: Only immediate children can access the shared state, which can limit the component hierarchy.
- Naming Clashes: There is a risk of naming conflicts with props when using techniques like React.cloneElement, making it difficult to manage large component trees.
- Complexity: Understanding and implementing the compound component pattern can be challenging, particularly for developers new to React.
Hooks Pattern:
The Hooks API provides a new way to write React components by permitting functional components to use state, lifecycle methods, context, and refs. Hooks were constructed to resolve limitations in class components and to boost code reuse and simplicity.
Pros:
- Cleaner Code: Hooks help organize code better, avoiding the boilerplate and complexity of lifecycle methods in class components.
- Boosted Reusability: Custom hooks allow the reuse of stateful logic across distinct components, limiting code duplication.
- Simplified Component Logic: Functional components with hooks are often easier to understand and maintain compared to class components.
Cons:
- Learning Curve: Developers need to follow specific rules and practices when using hooks, which can be challenging without proper tools and experience.
- Debugging Challenges: Debugging hooks can be harder compared to class components, as the component’s lifecycle is managed differently.
- Tooling Requirements: Effective use of hooks often requires additional tooling, such as linters, to enforce best practices and avoid common pitfalls.
Conclusion:
The React design patterns are among the most commonly utilized in development projects. By leveraging these patterns, you can elevate the capabilities of the React library. It is highly recommended to gain a thorough understanding of these patterns and apply them effectively. Doing so will enable you to create scalable and easily maintainable React applications.